基于.NET8实现WinFrom应用窗口自动缩放功能
1. git地址
基于.net8 的winfrom应用
gitee.com/mtoooo/win-from-window-adaptive-demo
2. 核心方法
winfrom页面控件列表及层级关系
顶部控件 panel1 = new Panel();
顶部控件添加
- 二级控件
groupBox1 = new GroupBox();
- 二级控件
groupBox2 = new GroupBox();
- 二级控件
groupBox3 = new GroupBox();
- 二级控件
groupBox4 = new GroupBox();
- 三级控件
label1 = new Label();
- 三级控件
label2 = new Label();
- 三级控件
控件核心方法及属性
父级节点绑定子级节点Parent.Controls.Add(Child)
子节点设置Anchor属性TopBottomLeftRight
多层遍历,根据窗口调整控件大小
int normalWidth = 0; int normalHeight = 0; //需要记录的控件的位置以及宽和高(X,Y,Widht,Height) DictionarynormalControl = new Dictionary (); private void Form1_Load(object sender, EventArgs e) { //记录相关对象以及原始尺寸 normalWidth = this.panel1.Width; normalHeight = this.panel1.Height; //通过父控件Panel进行控件的遍历 foreach (Control item in this.panel1.Controls) { normalControl.Add(item.Name, new Rect(item.Left, item.Top, item.Width, item.Height)); } } private void Form1_SizeChanged(object sender, EventArgs e) { //根据原始比例进行新尺寸的计算 int w = this.panel1.Width; int h = this.panel1.Height; foreach (Control item in this.panel1.Controls) { int newX = (int)(w * 1.00 / normalWidth * normalControl[item.Name].X); int newY = (int)(h * 1.00 / normalHeight * normalControl[item.Name].Y); int newW = (int)(w * 1.00 / normalWidth * normalControl[item.Name].Widht); int newH = (int)(h * 1.00 / normalHeight * normalControl[item.Name].Height); item.Left = newX; item.Top = newY; item.Width = newW; item.Height = newH; } }
3. 全部代码
Form1.cs
namespace WinFormsApp6 { public partial class Form1 : Form { public Form1() { InitializeComponent(); //this.Load += Form1_Load; //this.SizeChanged += Form1_SizeChanged; } private void button1_Click(object sender, EventArgs e) { } private void textBox2_TextChanged(object sender, EventArgs e) { } private void textBox1_TextChanged(object sender, EventArgs e) { } private void label20_Click(object sender, EventArgs e) { } private void comboBox6_SelectedIndexChanged(object sender, EventArgs e) { } private void comboBox4_SelectedIndexChanged(object sender, EventArgs e) { } private void groupBox4_Enter(object sender, EventArgs e) { } private void groupBox3_Enter(object sender, EventArgs e) { } private void groupBox5_Enter(object sender, EventArgs e) { } private void dataGridView1_CellContentClick(object sender, DataGridViewCellEventArgs e) { } private void groupBox1_Enter(object sender, EventArgs e) { } int normalWidth = 0; int normalHeight = 0; //需要记录的控件的位置以及宽和高(X,Y,Widht,Height) DictionarynormalControl = new Dictionary (); private void Form1_Load(object sender, EventArgs e) { //记录相关对象以及原始尺寸 normalWidth = this.panel1.Width; normalHeight = this.panel1.Height; //通过父控件Panel进行控件的遍历 foreach (Control item in this.panel1.Controls) { normalControl.Add(item.Name, new Rect(item.Left, item.Top, item.Width, item.Height)); } } private void Form1_SizeChanged(object sender, EventArgs e) { //根据原始比例进行新尺寸的计算 int w = this.panel1.Width; int h = this.panel1.Height; foreach (Control item in this.panel1.Controls) { int newX = (int)(w * 1.00 / normalWidth * normalControl[item.Name].X); int newY = (int)(h * 1.00 / normalHeight * normalControl[item.Name].Y); int newW = (int)(w * 1.00 / normalWidth * normalControl[item.Name].Widht); int newH = (int)(h * 1.00 / normalHeight * normalControl[item.Name].Height); item.Left = newX; item.Top = newY; item.Width = newW; item.Height = newH; } } private void button7_Click(object sender, EventArgs e) { } private void label15_Click(object sender, EventArgs e) { } } class Rect { public Rect(int x, int y, int w, int h) { this.X = x; this.Y = y; this.Widht = w; this.Height = h; } public int X { get; set; } public int Y { get; set; } public int Widht { get; set; } public int Height { get; set; } } }
Form1.Designer.cs
using System.Windows.Forms; namespace WinFormsApp6 { partial class Form1 { private System.ComponentModel.IContainer components = null; protected override void Dispose(bool disposing) { if (disposing && (components != null)) { components.Dispose(); } base.Dispose(disposing); } #region Windows Form Designer generated code private void InitializeComponent() { panel1 = new Panel(); groupBox1 = new GroupBox(); dataGridView1 = new DataGridView(); Column1 = new DataGridViewTextBoxColumn(); Column2 = new DataGridViewTextBoxColumn(); Column3 = new DataGridViewButtonColumn(); Column4 = new DataGridViewButtonColumn(); groupBox2 = new GroupBox(); label6 = new Label(); label5 = new Label(); label4 = new Label(); label3 = new Label(); progressBar2 = new ProgressBar(); label2 = new Label(); label1 = new Label(); progressBar1 = new ProgressBar(); groupBox5 = new GroupBox(); comboBox3 = new ComboBox(); comboBox7 = new ComboBox(); label21 = new Label(); comboBox6 = new ComboBox(); label20 = new Label(); comboBox5 = new ComboBox(); label19 = new Label(); comboBox4 = new ComboBox(); label18 = new Label(); label15 = new Label(); groupBox3 = new GroupBox(); groupBox1.SuspendLayout(); ((System.ComponentModel.ISupportInitialize)dataGridView1).BeginInit(); groupBox2.SuspendLayout(); groupBox5.SuspendLayout(); SuspendLayout(); // // panel1 // panel1.Anchor = AnchorStyles.Top | AnchorStyles.Bottom | AnchorStyles.Left | AnchorStyles.Right; panel1.Location = new Point(0, 0); panel1.Name = "panel1"; panel1.Size = new Size(200, 100); panel1.TabIndex = 0; // // groupBox1 // groupBox1.Anchor = AnchorStyles.Top | AnchorStyles.Bottom | AnchorStyles.Left | AnchorStyles.Right; groupBox1.Controls.Add(dataGridView1); groupBox1.Location = new Point(6, 12); groupBox1.Name = "groupBox1"; groupBox1.Size = new Size(800, 256); groupBox1.TabIndex = 0; groupBox1.TabStop = false; groupBox1.Text = "groupBox1"; groupBox1.Enter += groupBox1_Enter; // // dataGridView1 // dataGridView1.Anchor = AnchorStyles.Top | AnchorStyles.Bottom | AnchorStyles.Left | AnchorStyles.Right; dataGridView1.AutoSizeColumnsMode = DataGridViewAutoSizeColumnsMode.Fill; dataGridView1.BackgroundColor = SystemColors.Control; dataGridView1.ColumnHeadersHeightSizeMode = DataGridViewColumnHeadersHeightSizeMode.AutoSize; dataGridView1.Columns.AddRange(new DataGridViewColumn[] { Column1, Column2, Column3, Column4 }); dataGridView1.Location = new Point(6, 26); dataGridView1.Name = "dataGridView1"; dataGridView1.RowHeadersVisible = false; dataGridView1.RowHeadersWidth = 51; dataGridView1.Size = new Size(785, 224); dataGridView1.TabIndex = 0; dataGridView1.CellContentClick += dataGridView1_CellContentClick; // // Column1 // Column1.FillWeight = 50F; Column1.HeaderText = "序号"; Column1.MinimumWidth = 6; Column1.Name = "Column1"; // // Column2 // Column2.FillWeight = 150F; Column2.HeaderText = "文件路径"; Column2.MinimumWidth = 6; Column2.Name = "Column2"; // // Column3 // Column3.FillWeight = 50F; Column3.HeaderText = "选择"; Column3.MinimumWidth = 6; Column3.Name = "Column3"; // // Column4 // Column4.FillWeight = 50F; Column4.HeaderText = "删除"; Column4.MinimumWidth = 6; Column4.Name = "Column4"; // // groupBox2 // groupBox2.Anchor = AnchorStyles.Bottom | AnchorStyles.Left | AnchorStyles.Right; groupBox2.Controls.Add(label6); groupBox2.Controls.Add(label5); groupBox2.Controls.Add(label4); groupBox2.Controls.Add(label3); groupBox2.Controls.Add(progressBar2); groupBox2.Controls.Add(label2); groupBox2.Controls.Add(label1); groupBox2.Controls.Add(progressBar1); groupBox2.Location = new Point(6, 610); groupBox2.Name = "groupBox2"; groupBox2.Size = new Size(800, 211); groupBox2.TabIndex = 1; groupBox2.TabStop = false; groupBox2.Text = "groupBox3"; // // label6 // label6.Anchor = AnchorStyles.Top | AnchorStyles.Bottom | AnchorStyles.Left | AnchorStyles.Right; label6.AutoSize = true; label6.Location = new Point(512, 133); label6.Name = "label6"; label6.Size = new Size(22, 17); label6.TabIndex = 7; label6.Text = "25"; // // label5 // label5.Anchor = AnchorStyles.Top | AnchorStyles.Bottom | AnchorStyles.Left | AnchorStyles.Right; label5.AutoSize = true; label5.Location = new Point(512, 47); label5.Name = "label5"; label5.Size = new Size(29, 17); label5.TabIndex = 6; label5.Text = "100"; // // label4 // label4.Anchor = AnchorStyles.Top | AnchorStyles.Bottom | AnchorStyles.Left | AnchorStyles.Right; label4.AutoSize = true; label4.Location = new Point(350, 133); label4.Name = "label4"; label4.Size = new Size(123, 17); label4.TabIndex = 5; label4.Text = "Percent Complete:"; // // label3 // label3.Anchor = AnchorStyles.Top | AnchorStyles.Bottom | AnchorStyles.Left | AnchorStyles.Right; label3.AutoSize = true; label3.Location = new Point(6, 133); label3.Name = "label3"; label3.Size = new Size(56, 17); label3.TabIndex = 4; label3.Text = "升级进度"; // // progressBar2 // progressBar2.Anchor = AnchorStyles.Top | AnchorStyles.Bottom | AnchorStyles.Left | AnchorStyles.Right; progressBar2.Location = new Point(6, 156); progressBar2.Name = "progressBar2"; progressBar2.Size = new Size(779, 26); progressBar2.TabIndex = 3; progressBar2.Value = 25; // // label2 // label2.Anchor = AnchorStyles.Top | AnchorStyles.Bottom | AnchorStyles.Left | AnchorStyles.Right; label2.AutoSize = true; label2.Location = new Point(350, 47); label2.Name = "label2"; label2.Size = new Size(123, 17); label2.TabIndex = 2; label2.Text = "Percent Complete:"; // // label1 // label1.Anchor = AnchorStyles.Top | AnchorStyles.Bottom | AnchorStyles.Left | AnchorStyles.Right; label1.AutoSize = true; label1.Location = new Point(6, 47); label1.Name = "label1"; label1.Size = new Size(56, 17); label1.TabIndex = 1; label1.Text = "下载进度"; // // progressBar1 // progressBar1.Anchor = AnchorStyles.Top | AnchorStyles.Bottom | AnchorStyles.Left | AnchorStyles.Right; progressBar1.Location = new Point(6, 70); progressBar1.Name = "progressBar1"; progressBar1.Size = new Size(779, 26); progressBar1.TabIndex = 0; progressBar1.Value = 100; // // groupBox5 // groupBox5.Anchor = AnchorStyles.Bottom | AnchorStyles.Left | AnchorStyles.Right; groupBox5.Controls.Add(comboBox3); groupBox5.Controls.Add(comboBox7); groupBox5.Controls.Add(label21); groupBox5.Controls.Add(comboBox6); groupBox5.Controls.Add(label20); groupBox5.Controls.Add(comboBox5); groupBox5.Controls.Add(label19); groupBox5.Controls.Add(comboBox4); groupBox5.Controls.Add(label18); groupBox5.Controls.Add(label15); groupBox5.Location = new Point(6, 294); groupBox5.Name = "groupBox5"; groupBox5.Size = new Size(800, 284); groupBox5.TabIndex = 10; groupBox5.TabStop = false; groupBox5.Text = "groupBox2"; groupBox5.Enter += groupBox5_Enter; // // comboBox3 // comboBox3.Anchor = AnchorStyles.Top | AnchorStyles.Bottom | AnchorStyles.Left | AnchorStyles.Right; comboBox3.FormattingEnabled = true; comboBox3.Items.AddRange(new object[] { "00 = Automatic", "01 = By Message" }); comboBox3.Location = new Point(132, 18); comboBox3.Name = "comboBox3"; comboBox3.Size = new Size(659, 25); comboBox3.TabIndex = 21; comboBox3.Text = "00 = Automatic"; // // comboBox7 // comboBox7.Anchor = AnchorStyles.Top | AnchorStyles.Bottom | AnchorStyles.Left | AnchorStyles.Right; comboBox7.FormattingEnabled = true; comboBox7.Items.AddRange(new object[] { "01 = Activate", "02 = Rollback", "03 = Revert" }); comboBox7.Location = new Point(132, 218); comboBox7.Name = "comboBox7"; comboBox7.Size = new Size(659, 25); comboBox7.TabIndex = 25; comboBox7.Text = "01 = Activate"; // // label21 // label21.Anchor = AnchorStyles.Top | AnchorStyles.Bottom | AnchorStyles.Left | AnchorStyles.Right; label21.AutoSize = true; label21.Location = new Point(19, 226); label21.Name = "label21"; label21.Size = new Size(84, 17); label21.TabIndex = 24; label21.Text = "SwicthType:"; // // comboBox6 // comboBox6.Anchor = AnchorStyles.Top | AnchorStyles.Bottom | AnchorStyles.Left | AnchorStyles.Right; comboBox6.FormattingEnabled = true; comboBox6.Items.AddRange(new object[] { "01 = Warning", "02 = Error", "03 = Info", "04 = Debug" }); comboBox6.Location = new Point(132, 169); comboBox6.Name = "comboBox6"; comboBox6.Size = new Size(659, 25); comboBox6.TabIndex = 23; comboBox6.Text = "01 = Warning"; comboBox6.SelectedIndexChanged += comboBox6_SelectedIndexChanged; // // label20 // label20.Anchor = AnchorStyles.Top | AnchorStyles.Bottom | AnchorStyles.Left | AnchorStyles.Right; label20.AutoSize = true; label20.Location = new Point(6, 176); label20.Name = "label20"; label20.Size = new Size(96, 17); label20.TabIndex = 22; label20.Text = "MPULoglevel:"; label20.Click += label20_Click; // // comboBox5 // comboBox5.Anchor = AnchorStyles.Top | AnchorStyles.Bottom | AnchorStyles.Left | AnchorStyles.Right; comboBox5.FormattingEnabled = true; comboBox5.Items.AddRange(new object[] { "00 = All", "01 = only FOTA log", "02 = only MCU log", "03 = only MPU log", "04 = only Ethernet log" }); comboBox5.Location = new Point(132, 118); comboBox5.Name = "comboBox5"; comboBox5.Size = new Size(659, 25); comboBox5.TabIndex = 21; comboBox5.Text = "00 = All"; // // label19 // label19.Anchor = AnchorStyles.Top | AnchorStyles.Bottom | AnchorStyles.Left | AnchorStyles.Right; label19.AutoSize = true; label19.Location = new Point(6, 126); label19.Name = "label19"; label19.Size = new Size(98, 17); label19.TabIndex = 20; label19.Text = "MPULogType:"; // // comboBox4 // comboBox4.Anchor = AnchorStyles.Top | AnchorStyles.Bottom | AnchorStyles.Left | AnchorStyles.Right; comboBox4.FormattingEnabled = true; comboBox4.Items.AddRange(new object[] { "00 = No Requirement", "01 = Warning", "02 = Error", "03 = Info", "04 = Debug" }); comboBox4.Location = new Point(132, 68); comboBox4.Name = "comboBox4"; comboBox4.Size = new Size(659, 25); comboBox4.TabIndex = 19; comboBox4.Text = "00 = No Requirement"; comboBox4.SelectedIndexChanged += comboBox4_SelectedIndexChanged; // // label18 // label18.Anchor = AnchorStyles.Top | AnchorStyles.Bottom | AnchorStyles.Left | AnchorStyles.Right; label18.AutoSize = true; label18.Location = new Point(14, 76); label18.Name = "label18"; label18.Size = new Size(91, 17); label18.TabIndex = 18; label18.Text = "LogRecType:"; // // label15 // label15.Anchor = AnchorStyles.Top | AnchorStyles.Bottom | AnchorStyles.Left | AnchorStyles.Right; label15.AutoSize = true; label15.Location = new Point(9, 26); label15.Name = "label15"; label15.Size = new Size(93, 17); label15.TabIndex = 16; label15.Text = "ActivateType:"; label15.Click += label15_Click; // // groupBox3 // groupBox3.Anchor = AnchorStyles.Top | AnchorStyles.Bottom | AnchorStyles.Right; groupBox3.Location = new Point(812, 12); groupBox3.Name = "groupBox3"; groupBox3.Size = new Size(667, 809); groupBox3.TabIndex = 5; groupBox3.TabStop = false; groupBox3.Text = "log box"; groupBox3.Enter += groupBox3_Enter; // // Form1 // AutoScaleDimensions = new SizeF(7F, 17F); AutoScaleMode = AutoScaleMode.Font; ClientSize = new Size(1482, 833); Controls.Add(groupBox5); Controls.Add(groupBox3); Controls.Add(groupBox2); Controls.Add(groupBox1); Name = "Form1"; StartPosition = FormStartPosition.CenterScreen; Text = "窗口自适应 Tool"; Load += Form1_Load; groupBox1.ResumeLayout(false); ((System.ComponentModel.ISupportInitialize)dataGridView1).EndInit(); groupBox2.ResumeLayout(false); groupBox2.PerformLayout(); groupBox5.ResumeLayout(false); groupBox5.PerformLayout(); ResumeLayout(false); } #endregion private Panel panel1; private GroupBox groupBox1; private GroupBox groupBox2; private GroupBox groupBox3; private DataGridView dataGridView1; private DataGridViewTextBoxColumn Column1; private DataGridViewTextBoxColumn Column2; private DataGridViewButtonColumn Column3; private DataGridViewButtonColumn Column4; private Label label2; private Label label1; private ProgressBar progressBar1; private Label label5; private Label label4; private Label label3; private ProgressBar progressBar2; private Label label6; private Label label15; private ComboBox comboBox3; private GroupBox groupBox5; private ComboBox comboBox4; private Label label18; private ComboBox comboBox5; private Label label19; private Label label20; private ComboBox comboBox6; private ComboBox comboBox7; private Label label21; } }
Program.cs
namespace WinFormsApp6 { internal static class Program { ////// The main entry point for the application. /// [STAThread] static void Main() { // To customize application configuration such as set high DPI settings or default font, // see https://aka.ms/applicationconfiguration. ApplicationConfiguration.Initialize(); Application.Run(new Form1()); } } }
4. 显示效果
以上就是基于.NET8实现WinFrom应用窗口自动缩放功能的详细内容,更多关于.NET8 WinFrom窗口自动缩放的资料请关注科站长其它相关文章!
您可能感兴趣的文章
- 03-31详解如何在.NET代码中使用本地部署的Deepseek语言模型
- 02-06.net core如何使用Nacos注册中心
- 01-28使用.NET8构建一个高效的时间日期帮助类
- 01-26.NET Core GC压缩(compact_phase)底层原理解析
- 01-24在ASP.NET中读写TXT文本文件的多种方法
- 01-24在ASP.NET中读写XML数据的多种方法
- 01-24.NET轻松实现Excel转PDF的三种方法详解
- 01-23.NET9 AOT部署方案详解
- 01-23.NET NativeAOT 用法指南
- 01-23iis部署前后端分离项目全过程(Vuet前端和.NET6后端)
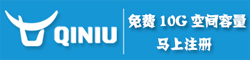
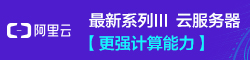
阅读排行
推荐教程
- 03-31详解如何在.NET代码中使用本地部署的Deepseek语言模型
- 11-23移动互联网广告有哪些模式?
- 11-22.net 应对网站访问压力的方案总结
- 11-22详解ASP.NET提取多层嵌套json数据的方法
- 11-23网站投放广告如何达到最好的效果
- 11-22.net 应对网站访问压力的方案总结
- 11-23网站打开速度慢解决办法
- 11-23草根站长为什么喜欢做门户站
- 11-22ASP.NET MVC分页问题解决
- 11-22ASP.NET编程简单实现生成静态页面的方法