JavaScript性能优化技术深入研究
目录
前言
javascript在浏览器中运行的性能,可以认为是开发者所面临的最严重的可用性问题。为了帮助大家有效的解决这个问题,今天给大家分享下这篇文章。
这个问题因为javascript的阻塞性而变得复杂,事实上,多数浏览器使用单一进程来处理用户界面和js脚本执行,所以同一时刻只能做一件事。js执行过程耗时越久,浏览器等待响应的时间越长 。
小知识:JavaScript性能优化涉及多个方面,包括代码执行效率、内存使用、DOM操作、网络请求等。通过合理的优化策略,可以显著提升应用的响应速度和用户体验。
性能分析工具实现
// 1. 性能计时器 class PerformanceTimer { constructor() { this.marks = new Map(); this.measures = new Map(); } mark(name) { this.marks.set(name, performance.now()); } measure(name, startMark, endMark) { const startTime = this.marks.get(startMark); const endTime = this.marks.get(endMark); if (startTime && endTime) { const duration = endTime - startTime; this.measures.set(name, duration); return duration; } return null; } getMeasure(name) { return this.measures.get(name); } clearMarks() { this.marks.clear(); } clearMeasures() { this.measures.clear(); } } // 2. 代码性能分析器 class CodeProfiler { constructor() { this.profiles = new Map(); } startProfile(name) { const startTime = performance.now(); const startMemory = performance.memory?.usedJSHeapSize; this.profiles.set(name, { startTime, startMemory, calls: 0, totalTime: 0, maxTime: 0 }); } endProfile(name) { const profile = this.profiles.get(name); if (!profile) return; const endTime = performance.now(); const endMemory = performance.memory?.usedJSHeapSize; const duration = endTime - profile.startTime; const memoryDiff = endMemory - profile.startMemory; profile.calls++; profile.totalTime += duration; profile.maxTime = Math.max(profile.maxTime, duration); profile.lastMemoryImpact = memoryDiff; } getProfile(name) { const profile = this.profiles.get(name); if (!profile) return null; return { ...profile, averageTime: profile.totalTime / profile.calls }; } getAllProfiles() { const results = {}; for (const [name, profile] of this.profiles) { results[name] = this.getProfile(name); } return results; } } // 3. 函数执行时间分析装饰器 function profileExecution(target, propertyKey, descriptor) { const originalMethod = descriptor.value; const profiler = new CodeProfiler(); descriptor.value = function(...args) { profiler.startProfile(propertyKey); const result = originalMethod.apply(this, args); profiler.endProfile(propertyKey); console.log(`Function ${propertyKey} profile:`, profiler.getProfile(propertyKey)); return result; }; return descriptor; }
代码优化技术
// 1. 循环优化 class LoopOptimizer { // 优化数组遍历 static optimizedForEach(array, callback) { const length = array.length; for (let i = 0; i < length; i++) { callback(array[i], i); } } // 分块处理大数组 static *chunkedProcess(array, chunkSize = 1000) { const length = array.length; for (let i = 0; i < length; i += chunkSize) { yield array.slice(i, Math.min(i + chunkSize, length)); } } // 使用Web Worker处理耗时操作 static createWorkerProcess(workerFunction) { const blob = new Blob([`(${workerFunction.toString()})()`], { type: 'application/javascript' }); return new Worker(URL.createObjectURL(blob)); } } // 2. 函数优化 class FunctionOptimizer { constructor() { this.cache = new Map(); } // 函数记忆化 memoize(fn) { return (...args) => { const key = JSON.stringify(args); if (this.cache.has(key)) { return this.cache.get(key); } const result = fn.apply(this, args); this.cache.set(key, result); return result; }; } // 函数防抖 debounce(fn, delay) { let timeoutId; return (...args) => { clearTimeout(timeoutId); timeoutId = setTimeout(() => fn.apply(this, args), delay); }; } // 函数节流 throttle(fn, limit) { let inThrottle; return (...args) => { if (!inThrottle) { fn.apply(this, args); inThrottle = true; setTimeout(() => inThrottle = false, limit); } }; } } // 3. DOM优化 class DOMOptimizer { constructor() { this.mutationObserver = null; this.virtualDOM = new Map(); } // 批量DOM更新 batchUpdate(updates) { const fragment = document.createDocumentFragment(); updates.forEach(update => { const element = this.createElement(update); fragment.appendChild(element); }); document.body.appendChild(fragment); } // 虚拟DOM实现 createElement(vnode) { if (typeof vnode === 'string') { return document.createTextNode(vnode); } const element = document.createElement(vnode.tag); for (const [key, value] of Object.entries(vnode.props || {})) { element.setAttribute(key, value); } (vnode.children || []).forEach(child => { element.appendChild(this.createElement(child)); }); return element; } // DOM变更监控 observeChanges(target, callback) { this.mutationObserver = new MutationObserver(callback); this.mutationObserver.observe(target, { childList: true, subtree: true, attributes: true }); } }
高级优化模式
// 1. 虚拟滚动实现 class VirtualScroller { constructor(container, items, itemHeight) { this.container = container; this.items = items; this.itemHeight = itemHeight; this.visibleItems = new Map(); this.scrollTop = 0; this.container.style.overflow = 'auto'; this.container.style.position = 'relative'; this.init(); } init() { // 设置容器高度 this.container.style.height = `${this.items.length * this.itemHeight}px`; // 监听滚动事件 this.container.addEventListener('scroll', this.onScroll.bind(this)); // 初始渲染 this.render(); } onScroll() { this.scrollTop = this.container.scrollTop; this.render(); } render() { const startIndex = Math.floor(this.scrollTop / this.itemHeight); const endIndex = Math.min( startIndex + Math.ceil(this.container.clientHeight / this.itemHeight), this.items.length ); // 移除不可见项 for (const [index, element] of this.visibleItems) { if (index < startIndex || index >= endIndex) { element.remove(); this.visibleItems.delete(index); } } // 添加可见项 for (let i = startIndex; i < endIndex; i++) { if (!this.visibleItems.has(i)) { const element = this.createItem(i); this.container.appendChild(element); this.visibleItems.set(i, element); } } } createItem(index) { const element = document.createElement('div'); element.style.position = 'absolute'; element.style.top = `${index * this.itemHeight}px`; element.style.height = `${this.itemHeight}px`; element.textContent = this.items[index]; return element; } } // 2. 资源预加载器 class ResourcePreloader { constructor() { this.cache = new Map(); this.loading = new Set(); } preload(resources) { resources.forEach(resource => { if (!this.cache.has(resource) && !this.loading.has(resource)) { this.loading.add(resource); const promise = this.loadResource(resource) .then(result => { this.cache.set(resource, result); this.loading.delete(resource); }) .catch(error => { console.error(`Failed to preload ${resource}:`, error); this.loading.delete(resource); }); return promise; } }); } loadResource(resource) { if (resource.endsWith('.js')) { return this.loadScript(resource); } else if (resource.endsWith('.css')) { return this.loadStyle(resource); } else if (/.(png|jpg|gif|svg)$/.test(resource)) { return this.loadImage(resource); } return Promise.reject(new Error('Unsupported resource type')); } loadScript(url) { return new Promise((resolve, reject) => { const script = document.createElement('script'); script.src = url; script.onload = () => resolve(script); script.onerror = reject; document.head.appendChild(script); }); } loadStyle(url) { return new Promise((resolve, reject) => { const link = document.createElement('link'); link.rel = 'stylesheet'; link.href = url; link.onload = () => resolve(link); link.onerror = reject; document.head.appendChild(link); }); } loadImage(url) { return new Promise((resolve, reject) => { const img = new Image(); img.src = url; img.onload = () => resolve(img); img.onerror = reject; }); } } // 3. Web Worker任务管理器 class WorkerTaskManager { constructor(workerScript) { this.worker = new Worker(workerScript); this.taskQueue = new Map(); this.taskId = 0; this.worker.onmessage = this.handleMessage.bind(this); this.worker.onerror = this.handleError.bind(this); } executeTask(task, data) { return new Promise((resolve, reject) => { const id = this.taskId++; this.taskQueue.set(id, { resolve, reject }); this.worker.postMessage({ id, task, data }); }); } handleMessage(event) { const { id, result, error } = event.data; const task = this.taskQueue.get(id); if (task) { if (error) { task.reject(error); } else { task.resolve(result); } this.taskQueue.delete(id); } } handleError(error) { console.error('Worker error:', error); } terminate() { this.worker.terminate(); this.taskQueue.clear(); } }
最佳实践建议
性能监控模式
// 1. 性能监控器 class PerformanceMonitor { constructor() { this.metrics = new Map(); this.thresholds = new Map(); } setThreshold(metric, value) { this.thresholds.set(metric, value); } recordMetric(metric, value) { if (!this.metrics.has(metric)) { this.metrics.set(metric, []); } const values = this.metrics.get(metric); values.push({ value, timestamp: Date.now() }); // 保持最近100个记录 if (values.length > 100) { values.shift(); } // 检查是否超过阈值 const threshold = this.thresholds.get(metric); if (threshold && value > threshold) { this.handleThresholdExceeded(metric, value, threshold); } } getMetricStats(metric) { const values = this.metrics.get(metric); if (!values || values.length === 0) { return null; } const numbers = values.map(v => v.value); return { average: numbers.reduce((a, b) => a + b) / numbers.length, max: Math.max(...numbers), min: Math.min(...numbers), current: numbers[numbers.length - 1] }; } handleThresholdExceeded(metric, value, threshold) { console.warn(`Performance threshold exceeded for ${metric}:`, { value, threshold, stats: this.getMetricStats(metric) }); } } // 2. 性能优化建议生成器 class PerformanceAdvisor { constructor() { this.rules = new Map(); this.initializeRules(); } initializeRules() { this.addRule('longTask', metrics => { if (metrics.taskDuration > 50) { return { severity: 'warning', message: '检测到长任务,考虑使用Web Worker或任务分割' }; } }); this.addRule('memoryLeak', metrics => { if (metrics.memoryGrowth > 10000000) { // 10MB return { severity: 'error', message: '检测到可能的内存泄漏' }; } }); this.addRule('domSize', metrics => { if (metrics.domNodes > 1000) { return { severity: 'warning', message: 'DOM节点数量过多,考虑使用虚拟滚动或延迟加载' }; } }); } addRule(name, checkFn) { this.rules.set(name, checkFn); } analyze(metrics) { const issues = []; for (const [name, checkFn] of this.rules) { const result = checkFn(metrics); if (result) { issues.push({ rule: name, ...result }); } } return issues; } } // 3. 性能报告生成器 class PerformanceReporter { constructor() { this.monitor = new PerformanceMonitor(); this.advisor = new PerformanceAdvisor(); } generateReport() { const metrics = { taskDuration: this.monitor.getMetricStats('taskDuration'), memoryGrowth: this.monitor.getMetricStats('memoryGrowth'), domNodes: this.monitor.getMetricStats('domNodes'), fps: this.monitor.getMetricStats('fps') }; const issues = this.advisor.analyze(metrics); return { timestamp: Date.now(), metrics, issues, recommendations: this.generateRecommendations(issues) }; } generateRecommendations(issues) { const recommendations = new Set(); issues.forEach(issue => { switch (issue.rule) { case 'longTask': recommendations.add('考虑使用Web Worker处理耗时任务'); recommendations.add('实现任务分割和调度'); break; case 'memoryLeak': recommendations.add('检查闭包和事件监听器'); recommendations.add('使用WeakMap/WeakSet存储对象引用'); break; case 'domSize': recommendations.add('实现虚拟滚动'); recommendations.add('使用文档片段批量更新DOM'); break; } }); return Array.from(recommendations); } }
结语
JavaScript性能优化是一个持续的过程,需要从多个维度进行考虑和实践。通过本文,我们学习了:
- 性能分析工具的实现
- 代码优化技术
- 高级优化模式
- 性能监控和优化建议
- 最佳实践和设计模式
学习建议:在实际开发中,要根据具体场景选择合适的优化策略。性能优化不是一蹴而就的,需要持续监控和改进。同时,过度优化可能会带来代码可维护性的问题,要在性能和可维护性之间找到平衡。
以上就是JavaScript性能优化技术深入研究的详细内容,更多关于JavaScript性能优化的资料请关注科站长其它相关文章!
上一篇:vue基于ElementUI动态设置表格高度的3种方法
栏 目:JavaScript
本文标题:JavaScript性能优化技术深入研究
本文地址:https://www.fushidao.cc/wangluobiancheng/3136.html
您可能感兴趣的文章
- 02-11js中基本事件的总结(onclick、onblur、onchange等)
- 02-11详解如何在Node.js中使用中间件处理请求
- 02-11Vue3中Provide和Inject的用法及工作原理详解
- 02-11Vue+vant实现图片上传添加水印
- 02-11快速解决 keep-alive 缓存组件中定时器干扰问题
- 02-11uniapp 使用 tree.js 解决模型加载不出来的问题及解决方法
- 02-11基于uniapp vue3 的滑动抢单组件实例代码
- 02-10JavaScript 中的 Map使用指南
- 02-10vue3中使用print-js组件实现打印操作步骤
- 02-10Vue 中v-model的完整用法及v-model的实现原理解析
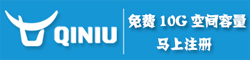
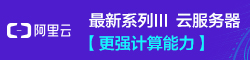
阅读排行
推荐教程
- 04-23JavaScript Array实例方法flat的实现
- 04-23THREE.JS使用TransformControls对模型拖拽的代码实例
- 04-23Vue3使用v-if指令进行条件渲染的实例代码
- 04-23vue3+ts项目搭建的实现示例
- 04-23JavaScript实现下载超大文件的方法详解
- 04-23vue如何使用pdf.js实现在线查看pdf文件功能
- 04-23vue.js调用python脚本并给脚本传数据
- 12-18使用JavaScript遍历输出页面中的所有元素的方法详解
- 04-23JS加密解密之保存到桌面书签
- 12-18Vue实现滚动加载更多效果的示例代码